The different operators in JavaScript
In programming, data is not only stored, but this data is worked with or modified and combined to obtain new data. In JavaScript, the so-called operators are used for this purpose.
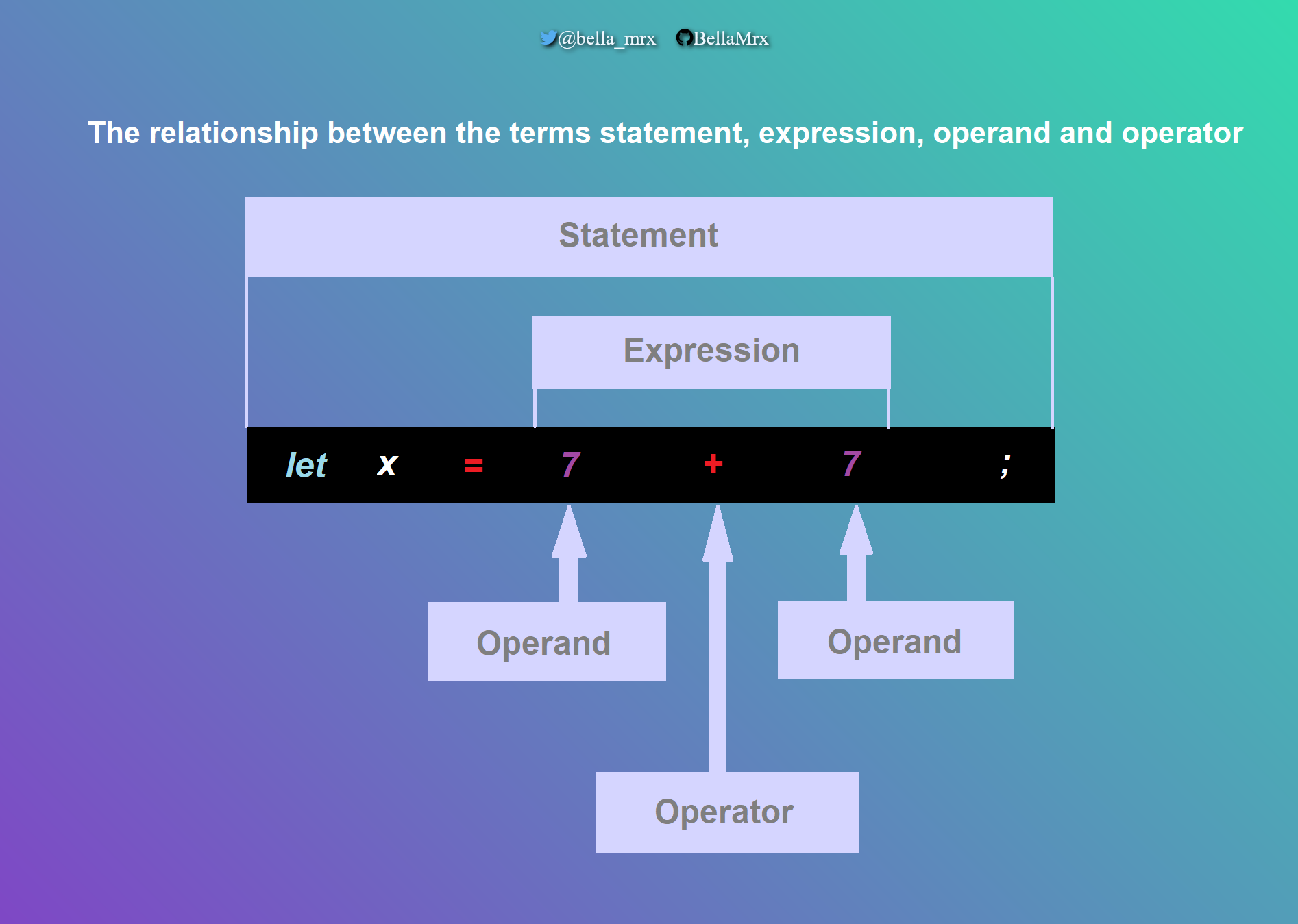
Both variables and simple values (literals) can be used as operands. Operators link the individual operands within an expression, whereby a distinction is made between the following types of operators depending on the number of operands:
- unary operator: An operator that refers to a single operand, e.g. the increment operator ++, this allows counter variables to be incremented directly by one counter++.
- binary operator: An operator that refers to two operands, e.g. the addition operator +, which can be used to add two numbers together x + y.
- ternary operator: This operator refers to three operands const variable = condition ? value1 : value2;
1. Operators for working with numbers
Arithmetic operators include operators for working and calculating with numbers: addition +, subtraction -, multiplication *, division / and the modulo %. There are also the increment and decrement operators, which add or subtract one to a number. Since ES2016, the exponential operator, which can be used to calculate powers, has also been included.
calculation type | operator | example | result of x |
---|---|---|---|
Addition | + | let x = 21 + 21 | 42 |
Subtraction | - | let x = 20 - 7 | 13 |
Multiplication | * | let x = 10 * 4 | 40 |
Division | / | let x = 40 / 4 | 10 |
Modulo | % | let x = 20 % 6 | 2 |
Increment | ++ | let x = 4; x++ | 5 |
Decrement | -- | let x = 4; x-- | 3 |
Power calculation | ** | let x = 4**4; | 256 |
The order in which the operators are evaluated when several arithmetic operators are used in an expression is simply a matter of remembering that multiplication and division are evaluated before addition and subtraction (as in mathematics: 4 + 4 * 5 = 20, (4 + 4) * 5 = 40).
2. Operators for simple assignment
It is often the case that you want to use a variable that already contains a number as an operand and at the same time assign the result of the respective operation to the variable as a new value, e.g. result = result + 1. As an alternative to this long notation, a short notation can be used for addition, subtraction, multiplication, division and the rest operator:
Complete Code - Examples/Part_20/main.js...
let result = 4;
result += 10; // The variable now has the value 14 ...
result -= 2; // ... now has the value 12 ...
result /= 3; // ... now has the value 4 ...
result *= 5; // ... now the value 20 ...
result %= 5; // ... and now the value 0.
Operator | Example | Long form |
---|---|---|
+= | result += 10 | result = result + 10 |
-= | result -= 10 | result = result - 10 |
*= | result *= 10 | result = result * 10 |
/= | result /= 10 | result = result / 10 |
%= | result %= 10 | result = result % 10 |
**= | result **= 10 | result = result ** 10 |
3. Operators for working with strings
Every now and then you have to combine two or more strings into a new string. The operator for merging two strings is the + operator in JavaScript, merging is also called concatenation.
Example:
Complete Code - Examples/Part_21/main.js...
const salutation = 'Professor'; // String 1
const name = 'Rick Sample'; // String 2
const message = salutation + name; // Concatenation
console.log(message); // output: ProfessorRick Sample
To insert a space after the salutation, this must simply be inserted into the concatenation. The + operator is used twice to insert a space between the variables salutation and name:
Complete Code - Examples/Part_22/main.js...
const salutation = 'Professor'; // String 1
const name = 'Rick Sample'; // String 2
const message = salutation + ' ' + name; // Concatenation plus space
console.log(message); // output: Professor Rick Sample
The only other operator that can be used with strings is the assignment operator +=:
Complete Code - Examples/Part_23/main.js...
let name = 'Rick Sample';
let message = 'Professor';
message += ' ';
message += name;
console.log(message); // output: Professor Rick Sample
4. Operators for working with Boolean values
The so-called logical operators are available for working with Boolean values. There are three operators in total:
Operator | Meaning |
---|---|
&& | logical AND |
|| | logical OR |
! | negation |
The AND operator and the OR operator are binary operators. The negation operator, on the other hand, is an unary operator, as it only expects a single operand.
The result of all Boolean operations is a Boolean value. The AND operator returns true if both operands are true, otherwise false. The OR operator returns true as soon as one of the two operands is true, and false only if both operands are false. The negation operator negates a Boolean value, i.e. if the operand is true, the operator returns a false. If the operand is false, the operator returns true.
Complete Code - Examples/Part_24/main.js...
const isLoggedIn = true;
const isAdmin = false;
const isLoggedInAndAdmin = isLoggedIn && isAdmin; // AND-operator
const isLoggedInOrAdmin = isLoggedIn || isAdmin; // OR-operator
const isLoggedOut = !isLoggedIn; // Negation
console.log(isLoggedInAndAdmin); // false
console.log(isLoggedInOrAdmin); // true
console.log(isLoggedOut); // false
Results for logical AND operations
Operand 1 | Operand 2 | Result |
---|---|---|
true | true | true |
true | false | false |
false | true | false |
false | false | false |
Results for logical OR operations
Operand 1 | Operand 2 | Result |
---|---|---|
true | true | true |
true | false | true |
false | true | true |
false | false | false |
Results for negation
Operand | Result |
---|---|
true | false |
false | true |
Boolean operators for non-Boolean operands
Boolean operators can also be used with operands that are not Boolean values. The JavaScript interpreter works according to the following rules:
The following applies to the logical AND operation:
- If the first operand evaluates to false, the first operand is returned. A value that evaluates to false is also called falsy (e.g. the number 0 or an empty string). In all other cases, the second operand is returned.
Results of the AND operation of non-boolean operands
Operand 1 | Operand 2 | Result |
---|---|---|
object | x | x |
true | x | x |
false | x | false |
x | null | null |
null | x | null |
x | NaN | NaN |
NaN | x | NaN |
x | undefined | undefined |
undefined | x | undefined |
Example:
Complete Code - Examples/Part_25/main.js...
const rick = {
firstName: 'Rick',
lastName: 'Sample'
};
const morty = {
firstName: 'Morty',
lastName: 'Sample'
};
const isRickAndMorty = rick && morty;
console.log(isRickAndMorty); // output: Object {firstName: "Morty",
// lastName: "Sample"}
console.log(false && 'Rick'); // output: false
console.log('Rick' && null); // output: null
console.log(null && 'Rick'); // output: null
The following applies to the logical OR operation:
- If the first operand evaluates to true, then the first operand is returned.
- In all other cases, the second operand is returned.
Results of the OR operation of non-Boolean operands
Operand 1 | Operand 2 | Result |
---|---|---|
object | x | object |
false | x | x |
x | null | x |
null | x | x |
null | null | null |
x | NaN | x |
NaN | x | x |
NaN | NaN | NaN |
x | undefined | x |
undefined | x | x |
undefined | undefined | undefined |
Example:
Complete Code - Examples/Part_26/main.js...
const rick = {
firstName: 'Rick',
lastName: 'Sample'
};
const morty = {
firstName: 'Morty',
lastName: 'Sample'
};
const isRickOrMorty = rick || morty;
console.log(isRickOrMorty); // output: Object {firstName: "Rick", lastName: "Sample"}
console.log(false || 'Rick'); // output: Rick
console.log('Rick' || null); // output: Rick
console.log(null || 'Rick'); // output: Rick
The following applies to negation:
- true is returned for an empty string, false for a non-empty string
- For all numbers except 0 (and Infinity) false is returned, for the number 0 true.
- For objects, false is returned
- For the values null, NaN and undefined, true is returned
Results of the negation of non-Boolean operands
Operand | Result |
---|---|
empty string | true |
non-empty string | false |
number 0 | true |
number not equal to 0 (and Infinity) | false |
object | false |
null | true |
NaN | true |
undefined | true |
Example:
Complete Code - Examples/Part_27/main.js...
const name = 'Rick Sample';
const emptyString = '';
console.log(!name); // output: false
console.log(!emptyString); // output: true
const amount = 0;
const age = 25;
console.log(!amount); // output: true
console.log(!age); // output: false
console.log(!null); // output: true
console.log(!NaN); // output: true
console.log(!undefined); // output: true
Nullish Coalecsing Operator
Another logical operator that was only introduced with ES2020 is the Nullish Coalecsing Operator consisting of two question marks ??. This ?? operator works in a similar way to the OR operator ||, with the difference that the ?? operator only returns the value of the right operand if the left operand is null or undefined.
Example:
Complete Code - Examples/Part_28/main.js...
const someNullValue = null;
const someUndefinedValue = undefined;
const someNumber = 0;
const someText = '';
const someBoolean = false;
// Left operand null --> Return of the right operand
const a = someNullValue ?? 'Default value for null';
console.log(a);
// output: Default value for null
// Left operand undefined --> Return of the right operand
const b = someUndefinedValue ?? 'Default value for undefined';
console.log(b);
// output: Default value for undefined
// Left operand 0 ("falsy") --> Return of the left operand
const c = someNumber ?? 42;
console.log(c);
// output: 0
// Left operand empty string ("falsy") --> Return of the left operand
const d = someText ?? 'Default value for empty string';
console.log(d);
// output: ''
// Left operand false --> Return of the left operand
const e = someBoolean ?? true;
console.log(e);
// output: false
const x = 420;
console.log({} ?? x); // {}
console.log(false ?? x); // false
console.log(x ?? null); // 420
console.log(null ?? x); // 420
console.log(null ?? null); // null
console.log(x ?? NaN); // 420
console.log(NaN ?? x); // NaN
console.log(x ?? undefined); // 420
console.log(undefined ?? x); // 420
console.log(undefined ?? undefined); // undefined
Results of the ??-linking of non-boolean operands
Operand 1 | Operand 2 | Result |
---|---|---|
object | x | object |
false | x | false |
x | null | x |
null | x | x |
null | null | null |
x | NaN | x |
NaN | x | x |
NaN | undefined | NaN |
x | NaN | x |
undefined | x | x |
undefined | undefined | undefined |
5. Operators for working with bits
With the so-called bitwise operations, it is possible to work with individual bits of values. However, this rarely occurs in practice.
Bitwise operators
Operator | Meaning |
---|---|
& | bitwise AND |
| | bitwise OR |
^ | bitwise exclusive OR |
~ | bitwise NOT |
<< | bitwise left shift |
>> | bitwise right shift |
>>> | bitwise sign-ignoring right shift |
Example:
Complete Code - Examples/Part_29/main.js...
let BYTE_A = 0b00000001; // Binary value 00000001, decimal value 1
// Bitwise left shift
BYTE_A = BYTE_A << 1; // Binary value 00000010, decimal value 2
BYTE_A = BYTE_A << 1; // Binary value 00000100, decimal value 4
BYTE_A = BYTE_A << 1; // Binary value 00001000, decimal value 8
BYTE_A = BYTE_A << 1; // Binary value 00010000, decimal value 16
// Bitwise right shift
BYTE_A = BYTE_A >> 1; // Binary value 00001000, decimal value 8
BYTE_A = BYTE_A >> 1; // Binary value 00000100, decimal value 4
BYTE_A = BYTE_A >> 1; // Binary value 00000010, decimal value 2
BYTE_A = BYTE_A >> 1; // Binary value 00000001, decimal value 1
let BYTE_B = 0b01000001; // Binary value 01000001, decimal value 65
// Bitwise AND
let BYTE_C = BYTE_A & BYTE_B; // Binary value 00000001, decimal value 1
// Bitwise OR
let BYTE_D = BYTE_A | BYTE_B; // Binary value 01000001, decimal value 65
// Bitwise exclusive OR
let BYTE_E = BYTE_A ^ BYTE_B; // Binary value 01000000, decimal value 64
6. Operators for comparing values
Comparing two values is probably the most frequently performed task in programming. There are various operators in JavaScript for comparing character strings, numbers and Boolean values:
Operator | Meaning | Description |
---|---|---|
== | equal | Compares two values and checks whether they are equal |
!= | unequal | Compares two values and checks whether they are unequal |
=== | strictly equal | Compares not only two values, but also the data types of the values. Only if the values and the data types are equal does it return true |
!== | strictly unequal | Compares not only two values, but also the data types of the values. If the values or the data types are not equal, this returns a true from |
< | smaller | Compares two values with each other and checks whether the left operand is smaller than the right operand |
> | greater | Compares two values with each other and checks whether the left operand is greater than the right operand |
<= | less than or equal to | Compares two values with each other and checks whether the left operand is less than or equal to the right operand |
>= | greater than or equal to | Compares two values with each other and checks whether the left operand is greater than or equal to the right operand |
The behavior of the different equality and inequality operators. There are two operators for each: == and === for testing for equality and != and !== for testing for inequality. The difference is that == and != only compare the two values as such and === and !== also compare the data types of the corresponding values. In other words, the operators == and != perform a non-strict comparison of two values, whereas the two operators === and !== perform a strict comparison of two values.
Example:
Complete Code - Examples/Part_30/main.js...
console.log(false == 0); // output: true
console.log(false == 1); // output: false
console.log(true == 1); // output: true
console.log(true == 0); // output: false
console.log("420" == 420); // output: true
console.log(false != 0); // output: false
console.log(false != 1); // output: true
console.log(true != 1); // output: false
console.log(true != 0); // output: true
console.log("420" != 420); // output: false
The == operator returns a true for the comparison false == 0, because the interpreter attempts to convert the second operand (the 0) into the data type of the first operand (a Boolean data type). Since 0 is converted to the boolean value false, the result of the comparison is a true. Conversely, if a 1 is converted to the Boolean value true, the result is false.
The two operators === and !== also check whether the data types of the two operands match:
Complete Code - Examples/Part_31/main.js...
console.log(false === 0); // output: false
console.log(false === 1); // output: false
console.log(true === 1); // output: false
console.log(true === 0); // output: false
console.log("420" === 420); // output: false
console.log(false !== 0); // output: true
console.log(false !== 1); // output: true
console.log(true !== 1); // output: true
console.log(true !== 0); // output: true
console.log("420" !== 420); // output: true
Here, the comparison of the values false and 0 results in the value false (unlike in the previous example), because the first operand is a Boolean value and the second operand is a number.
It is best to get into the habit of only using the strict comparison operator. This avoids automatic type conversion, which can often lead to errors in the program that are difficult to find.
The operators<, >, <= and >= work for numerical values in the same way as in mathematics. These operators work reliably when used with numerical values. If the operators are compared with a mixture of numerical values and other data types, the results are not always as expected due to the automatic type conversion.
7. Optional Chaining Operator
With ES2020, the so-called Optional Chaining Operator was added to the standard. When accessing a property, this operator is set directly after this property, which ensures that the next hierarchy level is only accessed if the corresponding property exists. If this is not the case, access is aborted:
Complete Code - Examples/Part_32/main.js...
const rick = {
firstName: 'Rick',
lastName: 'Sample',
contact: {
email: 'rick.sample@email.com'
}
};
const morty = {
firstName: 'Morty',
lastName: 'Sample',
};
// Access to nested property via
// Optional chaining operator:
console.log(rick.contact?.email); // output: rick.sample@email.com
console.log(morty.contact?.email); // output: undefined
// Alternatively also possible: Use of the
// operator on several hierarchy levels:
console.log(rick?.contact?.email); // output: rick.sample@email.com
console.log(morty?.contact?.email); // output: undefined
Before ES2020, this was very cumbersome in JavaScript: you had to check step by step for all properties on the way to the desired property whether they were also present in the hierarchy. Thanks to the optional chaining operator, this has become easier. The example Part_33/main.js shows again how it was done before ES2020.
The optional chaining operator also works for accessing methods. The operator is simply placed after the method call:
console.log(someObject.someFunction()?.someValue);
8. Logical Assignment Operators
The Logical Assignment Operators were included as standard with ES2021. These operators combine logical operators and assignment expressions. There are a total of three logical assignment operators, each of which works on two operands.
- ||=: The logical OR assignment is a combination of the logical || operator and the assignment operator =, it assigns the right operand to the left operand if the former has a falsy value, and is therefore a short form of the notation a || (a = b).
- &&=: The logical AND assignment operator, combines the logical && operator and the assignment operator = and only assigns the right operand to the left operand if the former has a truthy value, it is a short form of the notation a && (a = B).
- ??=: The logical nullish assignment, assigns the second operand to the first operand only if the first is null or undefined. It is a short form for the notation a ?? (a = b).
Example:
Complete Code - Examples/Part_35/main.js...
// logical OR assignment
let a1 = 5;
let a2 = null;
let a3 = false;
a1 ||= 7; // --> 5
a2 ||= 7; // --> 7
a3 ||= 7; // --> 7
console.log(`a1: ${a1}`); // "a1: 5"
console.log(`a2: ${a2}`); // "a2: 7"
console.log(`a3: ${a3}`); // "a3: 7"
// logical AND assignment
let b1 = 5;
let b2 = null;
let b3 = false;
b1 &&= 7; // --> 7
b2 &&= 7; // --> null
b3 &&= 7; // --> false
console.log(`b1: ${b1}`); // "b1: 7"
console.log(`b2: ${b2}`); // "b2: null"
console.log(`b3: ${b3}`); // "b3: false"
// logical nullish assignment
let c1 = 5;
let c2 = null;
let c3 = false;
c1 ??= 7; // --> 5
c2 ??= 7; // --> 7
c3 ??= 7; // --> false
console.log(`c1: ${c1}`); // "c1: 5"
console.log(`c2: ${c2}`); // "c2: 7"
console.log(`c3: ${c3}`); // "c3: false"
Initialization of object properties
Complete Code - Examples/Part_36/main.js...
const rick = {
firstName: 'Rick',
};
rick.firstName ||= 'Morty';
rick.lastName ||= 'Sample';
console.log(rick);
// { firstName: 'Rick', lastName: 'Sample' }
// The following was necessary before ES2021:
const jerry = {
firstName: 'Jerry',
};
jerry.firstName || (jerry.firstName = 'Morty');
jerry.lastName || (jerry.lastName = 'Sample');
console.log(jerry);
// { firstName: 'Jerry', lastName: 'Sample' }
The instruction rick.lastName ||= 'Sample'; ensures, for example, that the lastName property of the rick object is initialized with the value Sample if the property is not yet assigned. The other variant shows how it was done before ES2021.
9. Operators for special operations
There are other operators in JavaScript:
Operations | Operator | Description |
---|---|---|
Conditional operator | <condition> ? <value1> : <value2> | Ternary operator that returns one of two values depending on a condition |
Delete objects, object properties or elements within an array | delete | Allows elements to be deleted from arrays and object properties to be deleted from objects |
Existence of a property in an object | <property> in <object> | Checks whether an object has a property |
Type check | <object> instanceof <type> | Binary operator that checks whether an object is of a type |
Type determination | typeof <Operand> | Determines the data type of the operand. The operand can be an object, a string, a variable or a keyword such as true or false. Optionally, the operand can be specified in brackets. |
Related links: