Arrays
Arrays are nothing more than lists. Arrays can contain not just one, but several values. For example, in a shopping list, an array would be used for the representation, the individual entries, i.e. the items in the shopping cart, would then be the names (identification numbers). The easiest way to create an array in JavaScript is the so-called array literal notation. To define the beginning and end of an array, square brackets are used [ ]. The individual entries (or values or elements) in the square brackets are separated by commas.
1. Create and initialise arrays
Arrays can be created in JavaScript in two different ways. Either via a so-called constructor function or via the array literal notation.
An array is created as follows using the array constructor function:
const names = new Array();
If the number of values to be stored in the array is known in advance, this number can also be passed to the structure function as an argument, but this is not a must, as arrays grow dynamically.
const names = new Array(42);
If even the values to be stored in the array are known, these can also be passed directly as arguments:
const names = new Array('Rick', 'Morty', 'Summer');
Create arrays without the keyword new:
const names = Array('Rick', 'Morty', 'Summer');
If the constructor function Array() is called with a single value and this value is a number, this defines the length of the array. If it is called with two numbers as arguments, the length of the array is implicitly two and the array contains the two number elements.
The length of an array can be determined using the length property:
const names = new Array('Rick', 'Morty', 'Summer');
console.log(names.length); // Output: 3
The simplest way to create an array in JavaScript is the so-called rray literal notation. Square brackets [] are used to define the start and end of an array. The individual entries (or values or elements) in the square brackets are separated by commas.
const names = ['Rick', 'Morty', 'Summer']; // Create an array with specific values
const colors = []; // Create an empty array
The array literal notation can be conveniently combined with the object literal notation, for example to create an array of objects:
Complete Code - Examples/Part_152/main.js...
const contacts = [
{
firstName: 'Rick',
lastName: 'Sample',
email: 'rick.sample@mail.com'
},
{
firstName: 'Morty',
lastName: 'Sample',
email: 'morty.sample@mail.com'
},
{
firstName: 'Summer',
lastName: 'Sample',
email: 'summer.sample@mail.com'
}
];
Different types in arrays
It is also possible to store values of different types in a single array, i.e. character strings as well as numbers and booleans.
const values = [];
values[0] = 'Rick';
values[1] = 42;
values[2] = true;
An overview of the most important methods of arrays
Method | Description |
---|---|
concat() | Appends elements or arrays to an existing array |
filter() | Filters elements from the array on the basis of a filter criterion passed in the form of a function |
forEach() | Applies a passed function to each element in the array |
join() | Converts an array into a character string |
map() | Maps the elements of an array to new elements based on a transferred conversion function |
pop() | Removes the last element of an array |
push() | Inserts a new element at the end of the array |
reduce() | Combines the elements of an array into one value based on a passed function |
reverse() | Reverses the order of the elements in the array |
shift() | Removes the first element of an array |
slice() | Cuts individual elements out of an array |
splice() | Adds new elements at any position in the array |
sort() | Sorts the array, optionally based on a passed comparison function |
2. Access elements of an array
Like objects, arrays have key-value pairs, but the keys are index numbers. Counting starts at 0, i.e. the first element is at index 0, the second element at index 1 and so on.
Complete Code - Examples/Part_153/main.js...
const todoList = [
'Coding', // Element to Index 0
'Eating', // Element to Index 1
'Relaxing', // Element to Index 2
'Sleeping' // Element to Index 3
];
console.log(todoList[0]); // "Coding"
console.log(todoList[1]); // "Eating"
console.log(todoList[2]); // "Relaxing"
console.log(todoList[3]); // "Sleeping"
console.log(todoList[4]); // undefined
Individual elements in the array are accessed via this index. Square brackets [] are used to specify the index to be accessed. Accessing a non-existent Undex returns undefined as the return value.
A for loop can be used to iterate over the individual elements of an array:
Complete Code - Examples/Part_154/main.js...
for (let i = 0; i < todoList.length; i++) {
console.log(todoList[i]);
}
3. Add elements to an array
JavaScript provides various methods. These can be used to add elements at the beginning or end of an array or at any position in the array.
Add an element to the end of an array
The push() method is used to add one or more elements to the end of an array. The method returns the new length of the array.
Complete Code - Examples/Part_155/main.js...
const todoList = [];
let length;
length = todoList.push('Coding');
console.log(length); // output: 1
length = todoList.push('Eating');
console.log(length); // output: 2
length = todoList.push('Relaxing', 'Sleeping');
console.log(length); // output: 4
console.log(todoList); // output: ["Coding", "Eating", "Relaxing", "Sleeping"]
Here, a character string is first added twice to the array todoList via push(). The length of the array is assigned to the variable length after each call, which takes the values 1, 2 and 4 in succession.
Add an element at the beginning of the array
The unshift() method is used to add an element at the beginning of an array. This method changes the index assignment for all following elements. The return value is the new length of the array.
Complete Code - Examples/Part_156/main.js...
const todoList = [];
let length;
length = todoList.unshift('Coding');
console.log(length); // 1
length = todoList.unshift('Eating');
console.log(length); // 2
length = todoList.unshift('Relaxing', 'Sleeping');
console.log(length); // 4
console.log(todoList); // ["Relaxing", "Sleeping", "Eating", "Coding"]
Insert an element at any position in the array
To insert an element at an arbitrary position, the method splice() is used. The splice() method can also be used to delete or replace elements, more on this later.
Complete Code - Examples/Part_157/main.js...
const todoList = [
'Coding',
'Eating',
'Relaxing',
'Sleeping'
];
todoList.splice(
2, // Index from which to insert
0, // Number of elements to be deleted
'Shopping' // Element to be added
);
console.log(todoList);
// [
// "Coding",
// "Eating",
// "Shopping",
// "Relaxing",
// "Sleeping"
// ]
todoList.splice(
2, // Index from which to insert
0, // Number of elements to be deleted
'Napping', // Elements that are added ...
'Soccer training' // ... are to be
);
console.log(todoList);
/* [
"Coding",
"Eating",
"Napping",
"Soccer training",
"Shopping",
"Relaxing",
"Sleeping"
] */
Several arguments are passed to this method. The first argument represents the position from which the new elements are to be added. The second argument represents the number of elements to be deleted from the array. Initially, this argument is left at the value 0 so that splice() is only used to add elements. All further elements represent the elements to be added to the array.
4. Remove elements from an array
JavaScript also offers various options for removing an element from an array. The element can be removed at the beginning, at the end or a whole part in the middle of the array.
Remove the last array from an element
With the method pop() it is possible to remove the last element of an array. The method returns the removed element as the return value. If there is no element in the array, it returns the value undefined.
Complete Code - Examples/Part_158/main.js...
const todoList = [
'Coding',
'Eating',
'Relaxing',
'Sleeping'
];
const item1 = todoList.pop();
console.log(item1); // output: Sleeping
const item2 = todoList.pop();
console.log(item2); // output: Relaxing
const item3 = todoList.pop();
console.log(item3); // output: Eating
const item4 = todoList.pop();
console.log(item4); // output: Coding
const item5 = todoList.pop();
console.log(item5); // output: undefined
Remove the first element from an array
If the first element is to be removed from an array, the shift() method is used. The method returns the removed element as the return value. If there is no element in the array, it returns the value undefined.
Complete Code - Examples/Part_159/main.js...
const todoList = [
'Coding',
'Eating',
'Relaxing',
'Sleeping'
];
const item1 = todoList.shift();
console.log(item1); // output: Coding
const item2 = todoList.shift();
console.log(item2); // output: Eating
const item3 = todoList.shift();
console.log(item3); // output: Relaxing
const item4 = todoList.shift();
console.log(item4); // output: Sleeping
const item5 = todoList.shift();
console.log(item5); // output: undefined
Remove part of the elements from an array
Actually, splice() is used to add elements, for which the second argument is set to the value 0, and the elements to be added are then passed to it. However, this method can also be used to remove elements from an array, but the parameters are set differently for this. The first argument represents the position in the array from which elements are to be removed, the second argument the number of elements to be removed. No other arguments are passed as no arguments are to be added.
Complete Code - Examples/Part_160/main.js...
const todoList = [
'Coding',
'Eating',
'Relaxing',
'Sleeping'
];
const deletedTodos = todoList.splice(1, 2);
console.log(deletedTodos); // ["Eating", "Relaxing"]
console.log(todoList); // ["Coding", "Sleeping"]
Copy part of the elements from an array
Using the slice() method, it is possible to copy out some of the elements of an array. The return value is an array with copies of the corresponding elements. The original elements remain in the array. Two arguments can be passed to the method. The first argument specifies the position from which the extraction of the elements begins, the second optional argument specifies the position at which the extraction of the elements ends:
Complete Code - Examples/Part_161/main.js...
const todoList = [
'Coding',
'Eating',
'Relaxing',
'Sleeping'
];
const sliced1 = todoList.slice(1); // from second element
console.log(sliced1); // ["Eating", "Relaxing", "Sleeping"]
const sliced2 = todoList.slice(2); // from the third element
console.log(sliced2); // ["Relaxing", "Sleeping"]
const sliced3 = todoList.slice(0, 2); // first and second element
console.log(sliced3); // ["Coding", "Eating"]
const sliced4 = todoList.slice(2, 4); // third and fourth element
console.log(sliced4); // ["Relaxing", "Sleeping"]
// Origin array remains unchanged:
console.log(todoList); // ["Coding", "Eating", "Relaxing", "Sleeping"]
The method slice() can be called with positive numerical values as arguments, but also with negative numbers, which in turn has an effect on which part of the array is extracted:
Complete Code - Examples/Part_162/main.js...
const todoList = [
'Coding',
'Eating',
'Relaxing',
'Sleeping'
];
const sliced5 = todoList.slice(-2); // the last two elements
const sliced6 = todoList.slice(1, // second element from the beginning to ...
-1); // ... second element from the back
const sliced7 = todoList.slice(1, // second element from the front to ..
-2); // ... to third element from the end
const sliced8 = todoList.slice(1, // second element from the front to ...
-3); // ... fourth element from the end
console.log(sliced5); // ["Relaxing", "Sleeping"]
console.log(sliced6); // ["Eating", "Relaxing"]
console.log(sliced7); // ["Eating"]
console.log(sliced8); // []
5. Sorting arrays
Sorting data within an array is relatively simple. Two methods are available for this purpose. The reverse() and sort() methods. reverse() simply reverses the order of the elements in the array, the latter allows the elements to be sorted according to certain individual criteria.
Reverse the order of the elements in an array
If only the order of the elements in an array is to be reversed, the reverse() method is used. The method arranges the elements in reverse order and also returns the reversed array as the return value.
const names = ['Rick', 'Morty', 'Summer'];
names.reverse();
console.log(names); // output: Summer, Morty, Rick
The array names initially contains the character string Rick, Morty and Summer in this order. After calling the reverse() method, the character strings are in exactly the reverse order in the array, i.e. Summer, Morty and Rick.
Sort the elements in an array according to certain criteria
The sort() method offers more flexibility, as it allows you to optionally define your own sorting criterion that influences the order. The sort criterion is specified in the form of a comparison function, which is passed as an argument to the sort() method. The comparison function in turn has two parameters and is called internally in pairs for the values of the array when sort() is called. The return value of the function determines which of the two values is greater than the other:
- The return value -1 means that the first value is smaller than the second value.
- The return value 1 means that the first value is greater than the second value.
- The return value 0 means that both values are the same.
A comparison function that compares numerical values according to their size:
function compare(value1, value2) {
if (value1 < value2) {
return -1; // The first value is smaller than the second value.
} else if(value1 > value2) {
return 1; // The first value is greater than the second value.
} else {
return 0; // Both values are the same.
}
}
This comparison function can now be passed to the sort() method:
Complete Code - Examples/Part_163/main.js...
...
const values = [7, 5, 6, 9, 5, 2, 4];
values.sort(compare);
console.log(values); // 2, 4, 5, 5, 6, 7, 9
The initially unsorted array value is sorted after this method is called.
Sort objects in arrays
It becomes somewhat more complex when an array containing objects is sorted using the sort() method. For this purpose, the comparison functions compareByFirstName(), compareByLastName() and compareByEmail() have been defined, which compare individual elements with regard to various properties.
Complete Code - Examples/Part_164/main.js...
const contacts = [
{
firstName: 'Rick',
lastName: 'Sample',
email: 'rick.sample@mail.com'
},
{
firstName: 'Morty',
lastName: 'Sample',
email: 'cool.morty@mail.com'
},
{
firstName: 'Summer',
lastName: 'Sample',
email: 'summer@mail.com'
}
];
function compareByFirstName(contact1, contact2) {
return contact1.firstName.localeCompare(contact2.firstName);
}
function compareByLastName(contact1, contact2) {
return contact1.lastName.localeCompare(contact2.lastName);
}
function compareByEmail(contact1, contact2) {
return contact1.email.localeCompare(contact2.email);
}
contacts.sort(compareByFirstName); // Sort by first name
console.log(contacts[0].firstName); // Morty
console.log(contacts[1].firstName); // Rick
console.log(contacts[2].firstName); // Summer
contacts.sort(compareByLastName); // Sort by last name
console.log(contacts[0].firstName); // Morty
console.log(contacts[1].firstName); // Rick
console.log(contacts[2].firstName); // Summer
contacts.sort(compareByEmail); // Sort by e-mail address
console.log(contacts[0].firstName); // Morty
console.log(contacts[1].firstName); // Rick
console.log(contacts[2].firstName); // Summer
The localeCompare() method, which is available as standard for the character strings and takes into account country-specific settings for date and time formats and also the sorting of character strings, was used here within the comparison function. This method also returns one of three possible values. The value -1 in the event that the character string on which the method is called is smaller than the character string (in terms of alphabetical sorting) that was passed as an argument. The value 1 for the case that the character string is larger, and the value 0 for the case that both character strings are exactly the same size.
6. Use arrays as a stack
In programming, a stack refers to a data structure that works according to the so-called LIFO principle. LIFO means Last in First out and means that the last element added to the stack is the first element to be removed. As a rule, the method for adding elements is push() and the method for removing elements is pop().
Functionality of stacks:
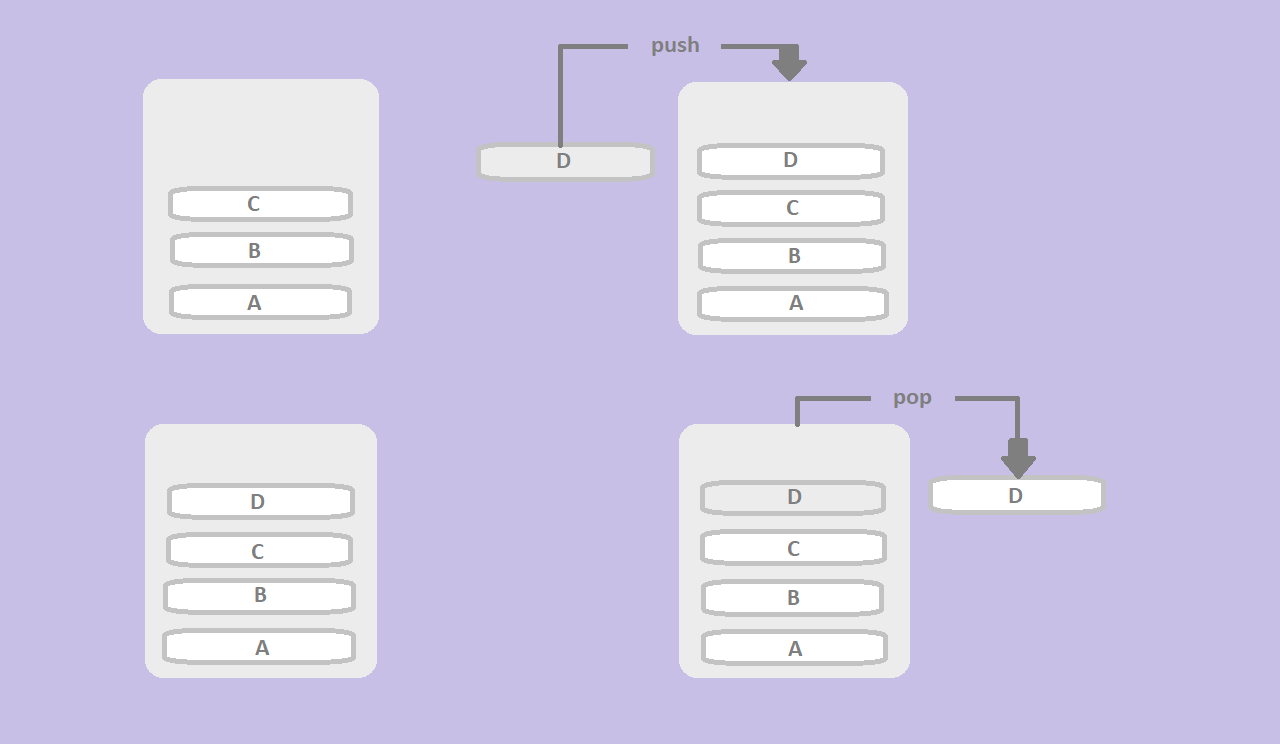
In JavaScript there is no explicit stack data structure in the form of a special object or something similar, but it is possible to emulate the behaviour described using arrays:
Complete Code - Examples/Part_165/main.js...
const stack = []; // Declaration of a normal array
stack.push(1); // Add an element ...
stack.push(2); // ... and another one ...
stack.push(3); // ... and one more ...
stack.push(4, 5, 6, 7, 8); // ... and several more in one go
console.log(stack.pop()); // The last element added, the 8th element, is returned
As an alternative to using push() and pop(), the shift() and unshift() methods can also be used to implement a stack. The stack then works the other way round. With unshift() elements are added at the front and with shift() the first element is removed.
7. Use arrays as a queue
Like the stack, a queue is also a data structure, but works in reverse. This means that a queue always returns the element that was added as the first element of the elements contained in the queue (FIFO principle, first in first out). In other words, the elements are added to the end of a queue, but removed from the beginning of the queue. As a rule, the corresponding methods are called enqueue() for adding elements and dequeue() for removing elements.
Functionality of queues:
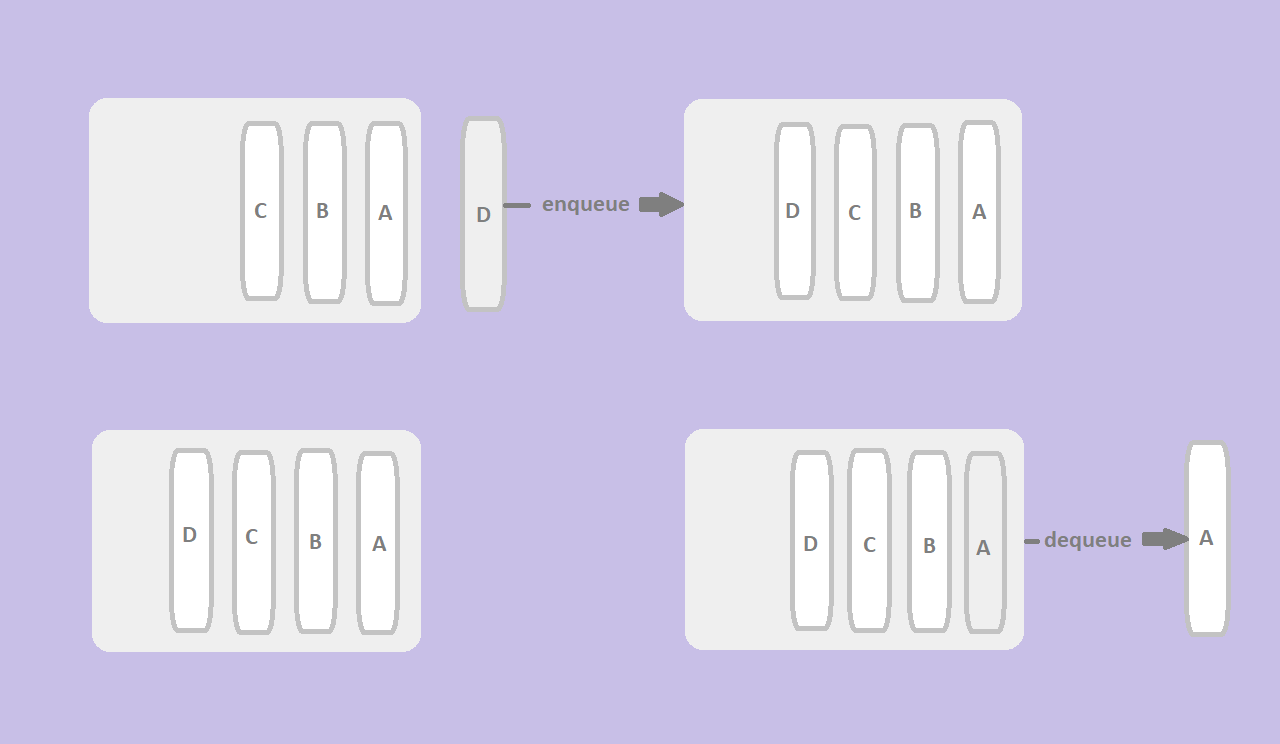
Queues can also be modelled using arrays in JavaScript:
Complete Code - Examples/Part_166/main.js...
const queue = []; // Declaration of a normal array
queue.push(1); // Add an element ...
queue.push(2); // ... and another one ...
queue.push(3); // ... and one more ...
queue.push(4, 5, 6, 7, 8); // ... and several more in one go
console.log(queue.shift()); // The first element added, the 1st element, is returned.
As an alternative to using push() and shift(), pop() and unshift() can also be used to implement a queue. The queue then works the other way round. With unshift() elements are added at the front, with pop() the last element is removed.
8. Find elements in arrays
The methods indexOf(), lastIndexOf() and find() are available to find elements in the array.
Search for elements from the begin of the array
The indexOf() method determines the position (index) for the transferred value at which it occurs for the first time within the array. If it is not contained in the array, the method returns the value -1. An optional second parameter can be used to control the index from which the search should start.
Complete Code - Examples/Part_167/main.js...
const transactions = [
-25.0, 399.99, -39.99, -32.50, 300, 490.99, -25, 300
];
console.log(transactions.indexOf(-25.0)); // output: 0
console.log(transactions.indexOf(400)); // output: -1
console.log(transactions.indexOf(300)); // output: 4
console.log(transactions.indexOf(300, 5)); // output: 7
Search for elements from the end of an array
The indexOf() method returns the first index at which the passed value was found in the array. Any subsequent elements are not taken into account. Alternatively, the lastIndexOf() method can be used, which searches from the end of an array and accordingly finds the last element of the transferred value in the array. Optionally, as with indexOf(), a second parameter can be used to define the index from which the search should start. Here too, the method returns the value -1.
Complete Code - Examples/Part_168/main.js...
const transactions = [
-25.0, 399.99, -39.99, -32.50, 300, 490.99, -25, 300
];
console.log(transactions.lastIndexOf(-25.0)); // output: 6
console.log(transactions.lastIndexOf(400)); // output: -1
console.log(transactions.lastIndexOf(300)); // output: 7
console.log(transactions.lastIndexOf(300, 5)); // output: 4
Additional method for arrays
ES2016 introduced the includes() method for arrays, which checks whether the transferred value is contained in the array or not and returns a corresponding Boolean value. Optionally, the index from which the element is to be searched for can be specified as the second parameter. This method checks whether an array contains a specific element.
Complete Code - Examples/Part_169/main.js...
[2, 4, 6].includes(4); // true
[1, 3, 7].includes(4); // false
['Rick', 'Morty'].includes('Summer'); // false
['Rick', 'Morty'].includes('Morty'); // true
[2, 4, 6, 420, 42, 84].includes(6, 2); // true
[2, 4, 6, 420, 42, 84].includes(6, 4); // false
Find elements for search criterion
In addition to the indexOf() and lastIndexOf() methods, there is also the findIndex() method and ES2016 also has the find() method. Both methods are passed a function as an argument, which is called for each element in the array and uses its Boolean return value to determine whether the respective element fulfils the search criterion or not. The findIndex() method returns the index of the first occurrence as the result, whereas the find() method returns the element itself.
Complete Code - Examples/Part_170/main.js...
const result = [2,3,4,5,6,7,2,3,4,5]
.find(element => element % 2 !== 0)
console.log(result); // output: 3
const result2 = [2,3,4,5,6,7,2,3,4,5]
.findIndex(element => element % 2 !== 0);
console.log(result2); // output: 1 --> Index
A function that returns the value true for odd numbers is passed as the search criterion here. Using the find() method with this function as an argument returns the value 3 because this is the first odd value in the array. The findIndex() method returns the value 1 accordingly, because the 3 occurs the first time at index 1 in the array.
9. Copy elements within an array
Some new methods for working with arrays have been added since version 6. One of these is the copyWithin() method, which can be used to copy elements within an array. The index to which the elements are to be copied, the index from which the elements are to be copied and, optionally, the index at which the copying of the elements ends are passed as arguments. The elements located at the position to which the elements are to be copied are replaced by these elements.
Complete Code - Examples/Part_171/main.js...
const todoList = [
'Coding',
'Eating',
'Relaxing',
'Sleeping'
];
todoList.copyWithin(
0, // Target start position to which the elements are copied
2, // Source start position from which copying starts
4 // Source end position up to which copying takes place
);
console.log(todoList);
// ["Relaxing", "Sleeping", "Relaxing", "Sleeping"]
Here in the array todoList, elements from index 2 to index 4 are copied to index 0 in the array. The two elements at index 0 (Coding) and index 1 (Eating) are replaced as required.
10. Convert arrays into strings
There are various options for converting arrays into a character string. On the one hand, the methods toString(), toLacaleString() and valueOf() can be used. On the other hand, arrays offer the join() method, which can be used to join individual elements of an array to form a character string. It is possible to specify a character string as a parameter that is to be used as a separator.
Complete Code - Examples/Part_172/main.js...
const names = ['Rick', 'Morty', 'Summer'];
const namesString = names.toString();
console.log(namesString); // output: Rick,Morty,Summer
const namesLocaleString = names.toLocaleString();
console.log(namesLocaleString); // output: Rick,Morty,Summer
const namesValue = names.valueOf();
console.log(namesValue); // output: ["Rick", "Morty", "Summer"]
const namesJoined = names.join('-');
console.log(namesJoined); // output: Rick-Morty-Summer
In addition to the array methods presented here, there are other methods, but these are among the most frequently used.
Related links: